A Python Health Check Running Daemon monitors system health and ensures services run smoothly. It automates health checks and alerts for potential issues.
A Python Health Check Running Daemon is essential for maintaining system reliability. This daemon continuously monitors various system parameters like CPU usage, memory consumption, and disk space. It ensures that all services operate within optimal limits. When it detects an anomaly, it triggers alerts or takes corrective actions.
This automation helps prevent downtime and maintains service efficiency. A health check daemon is critical for organizations relying on continuous service availability. Implementing such a daemon can significantly reduce manual monitoring efforts and quickly address potential problems. It is a proactive approach to system maintenance and reliability.
Introduction To Python Health Check
Python health check daemons are crucial for system stability. They monitor the system’s health, ensuring smooth operation. Understanding their role is essential for developers and IT professionals.
Importance Of System Stability
System stability is the backbone of any IT infrastructure. Unstable systems lead to downtime and loss of productivity. Regular health checks help prevent these issues.
Health checks identify potential problems before they cause system failures. This proactive approach saves time and resources. It ensures the system runs efficiently.
Role Of Health Check Daemons
Health check daemons are specialized programs. They run continuously in the background. These daemons monitor various system parameters.
- CPU usage
- Memory utilization
- Disk space
- Network connectivity
By tracking these metrics, daemons detect anomalies. They alert administrators to take corrective actions. This helps maintain system performance and reliability.
Python is a popular choice for writing health check daemons. It offers robust libraries and easy syntax. This makes development and maintenance simpler.
Here’s a simple example of a Python health check daemon:
import psutil
import time
def check_system_health():
while True:
cpu_usage = psutil.cpu_percent(interval=1)
mem = psutil.virtual_memory()
disk = psutil.disk_usage('/')
print(f"CPU Usage: {cpu_usage}%")
print(f"Memory Usage: {mem.percent}%")
print(f"Disk Usage: {disk.percent}%")
time.sleep(60)
if __name__ == "__main__":
check_system_health()
This script checks CPU, memory, and disk usage every minute. It prints the results to the console. This is a basic example, but it highlights the daemon’s role.
Setting Up The Environment
Setting up the environment is the first step to run a Python health check daemon. This involves installing necessary tools and configuring the Python environment. Below are the steps to get started.
Required Tools And Libraries
To set up the environment, you need some tools and libraries. Here is a list of what you need:
- Python 3.x: Ensure you have the latest version.
- pip: Python package installer.
- virtualenv: For creating isolated Python environments.
- psutil: For system and process utilities.
- logging: To log events for the daemon.
Configuring The Python Environment
After gathering the required tools, follow these steps to configure the Python environment:
- Install Python 3.x if not already installed.
- Use
pip
to install virtualenv: - Create a virtual environment:
- Activate the virtual environment:
- Install required libraries using
pip
:
pip install virtualenv
virtualenv venv
For Windows: venv\Scripts\activate
For macOS/Linux: source venv/bin/activate
pip install psutil
pip install logging
With the environment set up, you can now run your Python health check daemon. This setup ensures your daemon runs smoothly.
Core Components Of The Daemon
The Python Health Check Running Daemon ensures your system remains healthy. It continuously monitors key aspects of your system. Understanding its core components is crucial.
Monitoring System Metrics
The daemon tracks several system metrics to assess health.
- CPU Usage: Measures the percentage of CPU in use.
- Memory Usage: Tracks available and used memory.
- Disk Space: Monitors free and occupied disk space.
- Network Activity: Checks data sent and received.
It gathers these metrics at regular intervals. This helps in identifying any anomalies quickly. The daemon can alert you if any value exceeds the threshold.
Logging And Reporting
Logging and reporting are vital functions of the daemon. The daemon creates logs for all monitored metrics. These logs help in tracking historical data.
Metric | Logged Data |
---|---|
CPU Usage | Time-stamped CPU percentage |
Memory Usage | Time-stamped memory usage details |
Disk Space | Time-stamped disk space usage |
Network Activity | Time-stamped data transfer rates |
Reports are generated based on these logs. These reports provide insights into the system’s performance. You can view these reports to understand the system’s health trends.
The daemon can also send alerts if any metric goes beyond the set limit. This ensures prompt action to maintain system health.
# Example of logging CPU usage
import psutil
import logging
logging.basicConfig(filename='system_health.log', level=logging.INFO)
def log_cpu_usage():
cpu_usage = psutil.cpu_percent(interval=1)
logging.info(f'CPU Usage: {cpu_usage}%')
The above code logs CPU usage at regular intervals. Such logs are essential for maintaining system health.
Implementing The Health Check Daemon
Implementing a health check daemon for your Python applications is crucial. It ensures your services are running smoothly. This section will guide you step-by-step.
Writing The Core Script
Begin by writing the core script. This script will check the health of your services. The script should be simple and efficient.
Here’s a basic example:
import os
import time
def check_health():
response = os.system("ping -c 1 google.com")
if response == 0:
print("Service is up!")
else:
print("Service is down!")
if __name__ == "__main__":
while True:
check_health()
time.sleep(60) # Check every 60 seconds
This script pings Google to check internet connectivity. Adjust the script to suit your needs.
Scheduling The Daemon
After writing the script, schedule it to run as a daemon. This will automate the health checks.
Use cron jobs on Unix-based systems.
Example cron job entry:
/usr/bin/python3 /path/to/your_script.py
This entry runs your script every minute. Adjust the timing as needed.
On Windows, use Task Scheduler:
- Open Task Scheduler.
- Create a new task.
- Set the trigger to your desired time interval.
- Set the action to run your Python script.
These methods ensure your daemon runs continuously. Monitoring your services becomes automated and reliable.
Handling Alerts And Notifications
When running a Python Health Check Daemon, handling alerts and notifications is crucial. It ensures prompt action when issues arise. This section will discuss how to set alert thresholds and the various notification mechanisms available.
Setting Alert Thresholds
Setting alert thresholds helps in identifying problems early. These thresholds define the limits of acceptable performance or behavior. Exceeding these limits triggers an alert.
- Define CPU usage limits.
- Set memory consumption boundaries.
- Monitor disk space utilization.
- Establish network latency thresholds.
Use the following code snippet to set thresholds:
thresholds = {
'cpu_usage': 80,
'memory_usage': 75,
'disk_space': 90,
'network_latency': 100
}
Notification Mechanisms
Choosing the right notification mechanisms ensures timely alerts. Different methods can be used based on preference and criticality.
Mechanism | Advantages | Disadvantages |
---|---|---|
Widespread use | Can be delayed | |
SMS | Quick delivery | Costly for bulk |
Push Notifications | Immediate attention | Requires app installation |
Webhook | Custom integrations | Complex setup |
Use the following code snippet to send an email alert:
import smtplib
def send_email(subject, body, to):
message = f"Subject: {subject}\n\n{body}"
with smtplib.SMTP("smtp.example.com") as server:
server.login("user@example.com", "password")
server.sendmail("from@example.com", to, message)
Selecting the right combination of alert thresholds and notification mechanisms enhances system reliability.
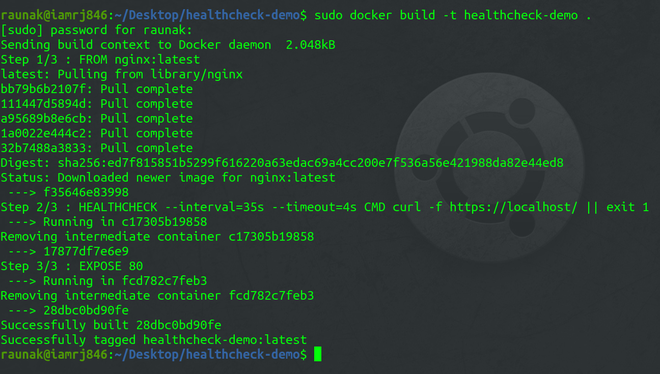
Testing And Validation
The Testing and Validation phase is crucial for a Python health check running daemon. This ensures the daemon operates reliably under real-world conditions. In this section, we will explore how to simulate system load and validate alert accuracy.
Simulating System Load
To ensure the daemon can handle high traffic, simulate system load. You can create artificial workloads to test the daemon’s performance.
- Use tools like Stress or Apache JMeter to generate load.
- Monitor CPU, memory, and disk usage during the simulation.
- Check the daemon’s response time and error rates.
Incorporate different types of load scenarios. This includes both high and low traffic conditions. This helps in understanding how the daemon behaves under various loads.
Validating Alert Accuracy
Ensuring the accuracy of alerts is vital. False alerts can be annoying and misleading. Here’s how to validate alert accuracy:
- Trigger known issues to test alert generation.
- Check if the daemon accurately detects and reports these issues.
- Review the logs for any missed alerts or false positives.
Use a combination of automated tests and manual checks to validate alert accuracy. This ensures that the daemon can reliably detect real issues.
Regularly update the alert criteria to match evolving system conditions. This helps in maintaining long-term accuracy and reliability.
Optimizing Performance
Optimizing the performance of your Python Health Check Running Daemon is crucial. It ensures your system runs smoothly and efficiently. Let’s dive into ways to enhance performance.
Reducing Resource Usage
Reducing resource usage is key to optimizing performance. Here are some tips:
- Limit the frequency of health checks. Run checks at optimal intervals.
- Use lightweight checks that consume fewer resources.
- Implement caching to store frequent check results.
Consider the following Python snippet for efficient resource usage:
import time
def health_check():
# Perform health check
pass
while True:
health_check()
time.sleep(60) # Run check every 60 seconds
Improving Response Time
Improving response time is equally important. Faster checks lead to quicker issue identification. Follow these steps:
- Optimize your code by removing unnecessary operations.
- Use asynchronous programming to handle multiple checks.
- Minimize I/O operations within health checks.
Here’s a sample code snippet for asynchronous health checks:
import asyncio
async def health_check():
# Perform health check
pass
async def main():
while True:
await health_check()
await asyncio.sleep(60) # Run check every 60 seconds
asyncio.run(main())
By following these tips, you can ensure your Python Health Check Running Daemon runs efficiently. This leads to better performance and quicker issue detection.
Deploying In Production
Deploying a Python Health Check Running Daemon in a production environment requires careful planning. Ensuring the daemon performs optimally and reliably is crucial. This section covers best practices and real-time monitoring techniques.
Best Practices For Deployment
Follow these best practices to deploy your Python Health Check Running Daemon successfully:
- Use Virtual Environments: Isolate dependencies to avoid conflicts.
- Automate Deployment: Use tools like Docker for consistent environments.
- Configuration Management: Employ tools like Ansible for maintaining configuration.
Monitoring In Real-time
Real-time monitoring ensures that your daemon remains healthy and responsive. Implement these strategies:
- Log Aggregation: Use tools like ELK Stack to collect and analyze logs.
- Health Dashboards: Create dashboards with Grafana to visualize health metrics.
- Alerting Systems: Set up alerts with Prometheus to detect issues quickly.
Tool | Purpose |
---|---|
Docker | Containerization for consistent deployment environments |
ELK Stack | Log aggregation and analysis |
Grafana | Creating health dashboards |
Prometheus | Setting up alerting systems |
Use these tools and practices to keep your Python Health Check Running Daemon robust and reliable in production.
Future Enhancements
The Python Health Check Running Daemon is already a powerful tool. Yet, continuous improvements can make it even more effective. Below are some exciting future enhancements that can elevate its capabilities.
Advanced Monitoring Features
Adding advanced monitoring features can drastically improve the daemon’s utility. These features will help in detailed health checks and diagnostics.
- Real-time Analytics: Track metrics in real-time for quick issue detection.
- Customizable Alerts: Set personalized alerts for specific events or thresholds.
- Historical Data: Store and analyze data over time for trend analysis.
These advanced features provide deeper insights into system health. They make the daemon a comprehensive tool for monitoring and diagnostics.
Integration With Other Tools
Integrating the daemon with other tools can enhance its functionality. Seamless integration can offer a holistic approach to system health management.
Tool | Benefits |
---|---|
Grafana | Visualize health metrics with dynamic dashboards. |
Slack | Receive health alerts directly in your Slack channels. |
Prometheus | Enable advanced scraping and alerting capabilities. |
These integrations offer more control and visibility. They allow for a more integrated and efficient monitoring system.
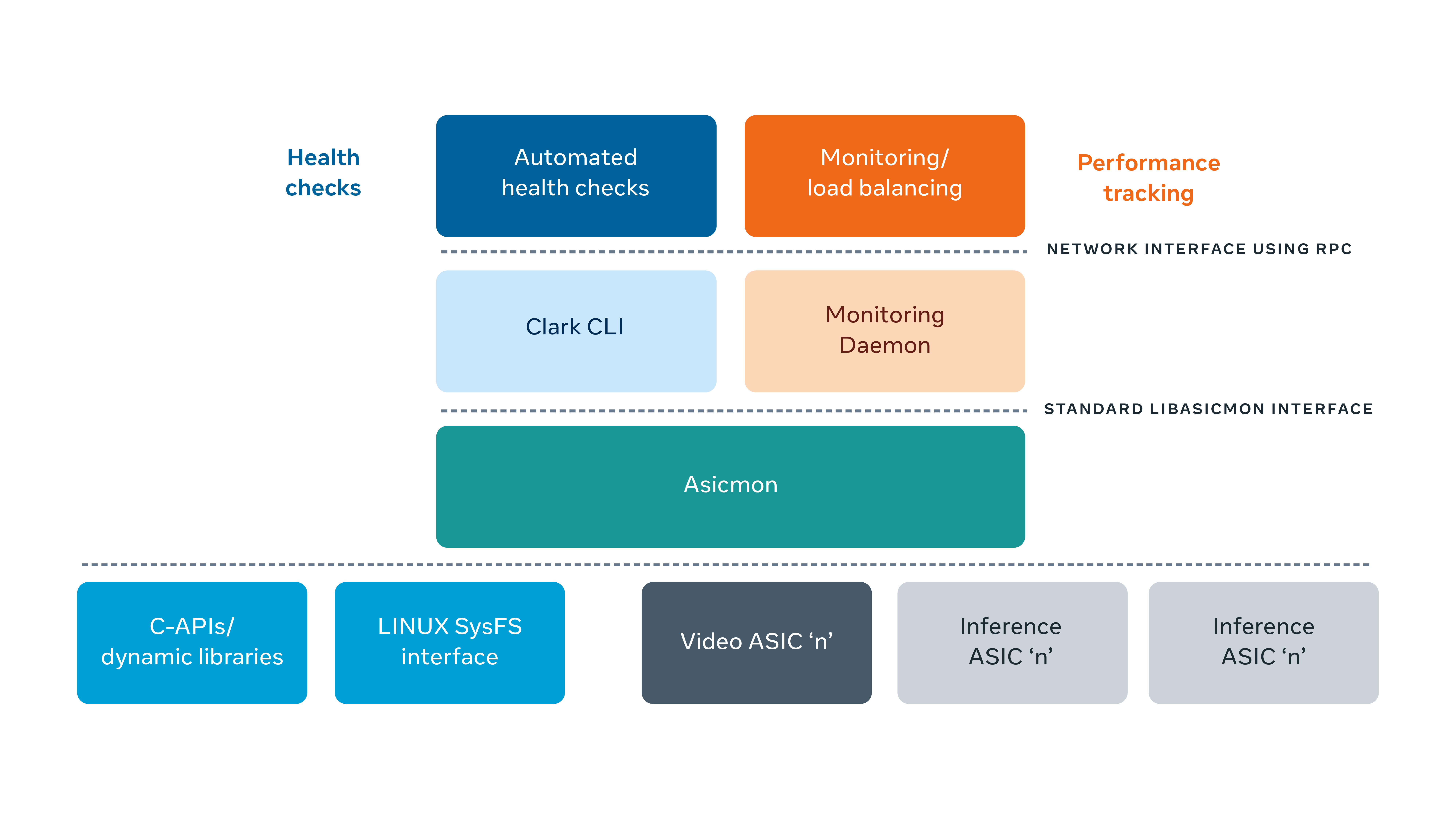

Frequently Asked Questions
What Is A Python Health Check Daemon?
A Python health check daemon is a background service. It monitors the health and performance of applications. It ensures the system runs smoothly.
How Does A Health Check Daemon Work?
A health check daemon runs periodic checks. It collects data on system performance and resource usage. It alerts if issues are found.
Why Use A Python Health Check Daemon?
Using a Python health check daemon ensures your application runs efficiently. It helps in early detection of issues. It also improves system reliability.
Can A Health Check Daemon Prevent Downtime?
Yes, a health check daemon can prevent downtime. It identifies problems early. It allows for quick resolution before major failures occur.
Conclusion
A Python Health Check Running Daemon ensures your applications stay reliable and efficient. It continuously monitors system health, providing crucial insights. Implementing this tool can significantly reduce downtime and improve performance. Invest time in creating a robust health check daemon to maintain optimal functionality and ensure smooth operations for your software applications.
Leave a Reply
You must be logged in to post a comment.