To force a Git pull, use `git fetch –all` followed by `git reset –hard origin/your-branch`. This ensures your local branch matches the remote branch.
Git is a powerful version control system used by developers. It helps manage code changes across multiple contributors. Sometimes, you need to force a Git pull to sync your local repository with the remote one. This is crucial when conflicts arise or when your local changes need to be discarded.
The process ensures that your local branch mirrors the remote branch exactly. It’s a straightforward yet critical operation for maintaining code consistency. Using the right commands can save time and prevent errors. By mastering this, you ensure smoother collaboration and code management.
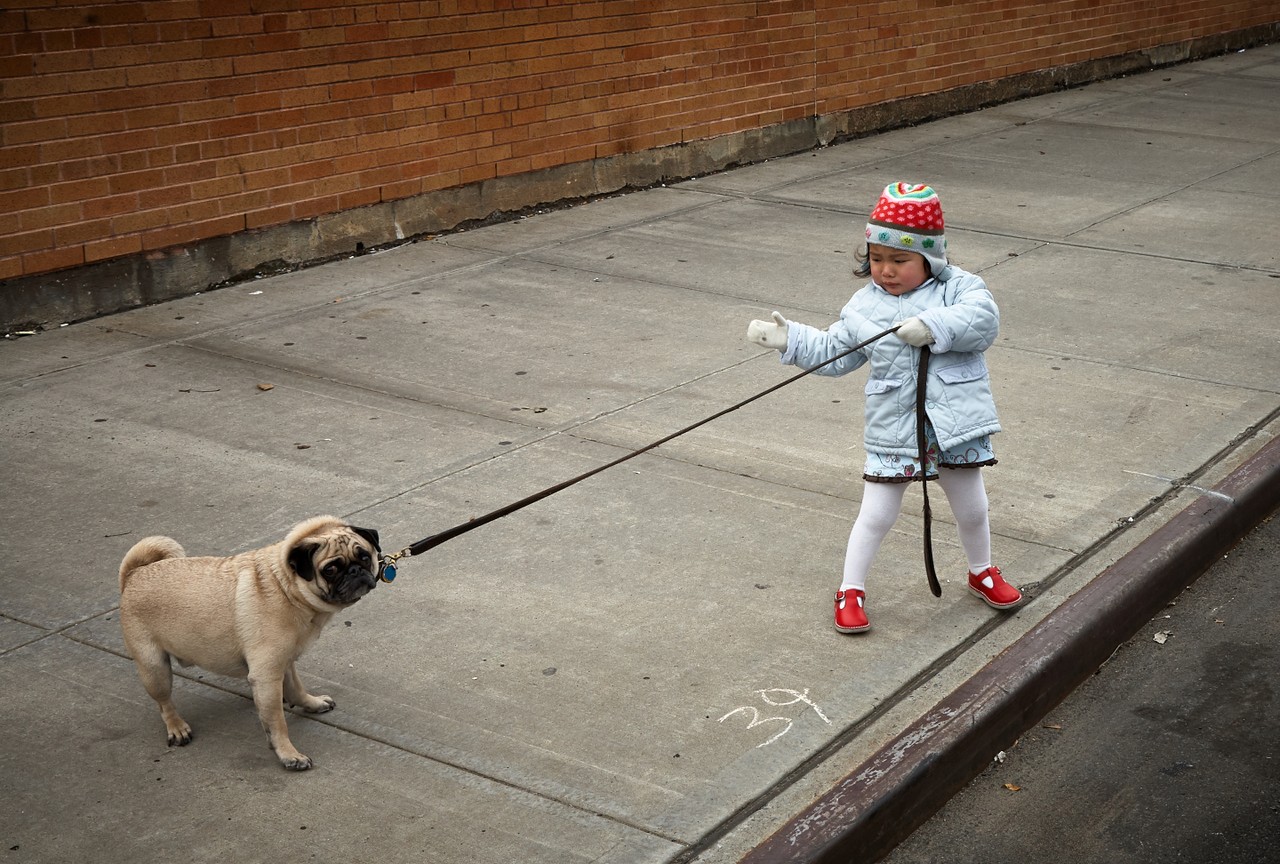
Introduction To Git Pull
Git is a powerful tool for version control. It helps developers collaborate. Git pull is a command used to update your local repository. It fetches and merges changes from a remote repository.
Understanding how to force a Git pull is essential. It ensures your local code is always up-to-date. This command keeps your work in sync with the team’s changes.
Purpose And Benefits
The main purpose of a Git pull is to update your local repository. It does this by fetching changes from a remote repository. Then it merges those changes into your local branch.
Here are some benefits:
- Synchronization: Keeps your local code in sync with the remote repository.
- Collaboration: Makes it easier to work with other team members.
- Efficiency: Reduces the risk of conflicts by ensuring everyone has the latest code.
Common Use Cases
Git pull is used in various scenarios. Here are some common use cases:
- Start of the Day: Update your local repository at the start of your workday.
- Before Pushing Code: Ensure your changes don’t conflict with others.
- After a Merge: Keep your branch updated after merging a pull request.
The git pull
command is simple but powerful. It ensures you always work with the latest code.
Basic Git Pull Command
The basic Git pull command is essential for syncing your local repository with a remote one. It fetches and integrates changes from a remote repository to your local branch. This ensures you have the most up-to-date version of the code.
Syntax And Parameters
The syntax for the basic Git pull command is straightforward:
git pull [options] [repository] [refspec]
Here is a breakdown of the parameters:
- options: Additional command-line options.
- repository: The remote repository name.
- refspec: The branch or tag to pull.
Here are some common options:
Option | Description |
---|---|
--rebase | Rebase the current branch on top of the upstream branch. |
--all | Fetch all remotes. |
Examples
Below are some examples of using the git pull command:
Pull from the default remote repository:
git pull
Pull from a specific remote repository named
origin
:git pull origin
Pull and rebase the current branch:
git pull --rebase
Using these commands keeps your local repository up-to-date. This helps to avoid conflicts and ensures smoother collaboration with your team.
Forcing A Git Pull
Sometimes, you need to force a Git pull to ensure your local repository matches the remote one. This can be necessary when dealing with conflicts or outdated files. Forcing a pull can help keep your codebase clean and up-to-date.
When To Use Force
Forcing a Git pull should be used carefully. It is best used in specific scenarios:
- Your local branch is significantly behind the remote branch.
- You have local changes that are not important to keep.
- There are conflicts that cannot be resolved manually.
To force a Git pull, use the command:
git fetch origin
git reset --hard origin/master
This command will fetch the latest changes and reset your local branch to match the remote branch.
Risks And Precautions
Forcing a Git pull can be risky. It can lead to data loss if not done correctly. Always take precautions:
- Backup your work before forcing a pull.
- Communicate with your team to avoid conflicts.
- Review changes before applying them.
Understanding the risks ensures you use force pulls responsibly. This keeps your workflow smooth and prevents data loss.
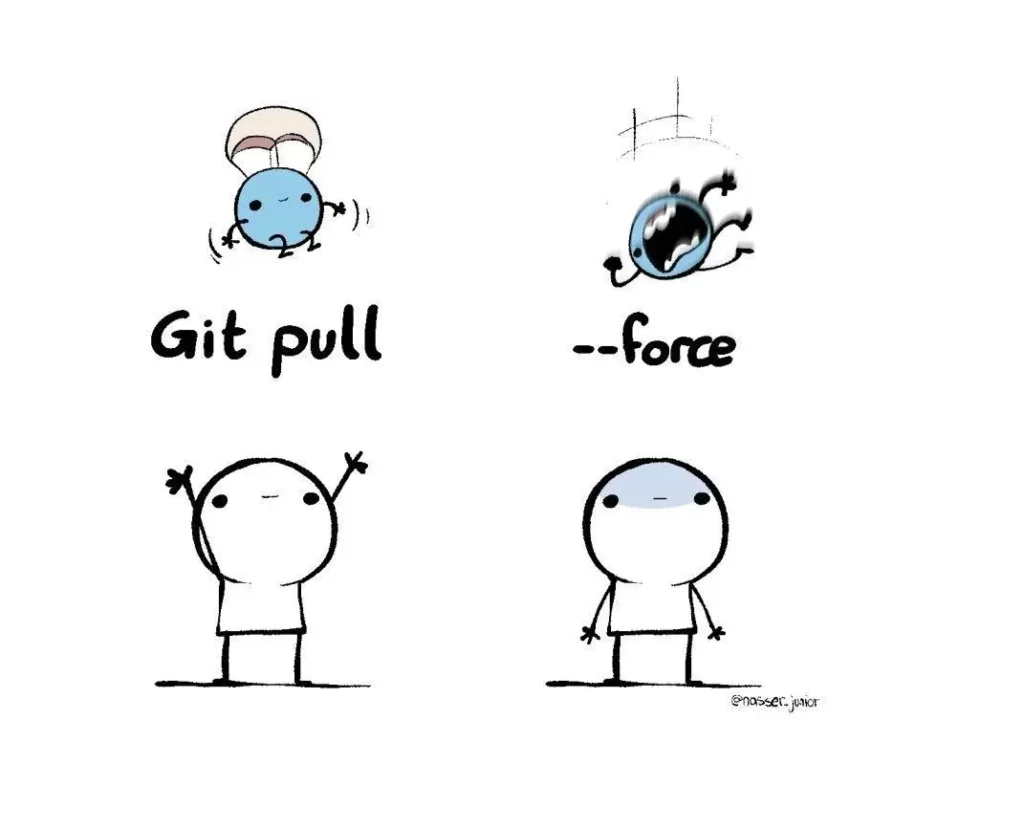
Steps To Force A Git Pull
Forcing a Git pull is sometimes necessary when you need to sync your local repository with the remote repository. This ensures that your local codebase is up-to-date. Below are the steps to force a Git pull.
Preparation
Before you force a Git pull, ensure you have the latest changes from the remote repository. This prevents conflicts and data loss.
- Open your terminal or command prompt.
- Navigate to your project directory using the
cd
command. - Check the status of your local repository using
git status
.
Example:
cd path/to/your/project
git status
Executing The Command
To force a Git pull, use the following command:
git fetch origin
git reset --hard origin/master
Explanation of the commands:
Command | Description |
---|---|
git fetch origin | Fetches the latest changes from the remote repository. |
git reset --hard origin/master | Resets your local repository to match the remote repository. |
After executing these commands, your local repository will be in sync with the remote repository. This is useful if you encounter conflicts or errors.
Always be cautious when using the git reset –hard command. It will overwrite your local changes.
Handling Conflicts
Handling conflicts during a Git pull is crucial. It ensures a smooth workflow. When multiple developers work on a project, conflicts can occur. These conflicts need prompt resolution to keep the project moving forward.
Identifying Conflicts
Identifying conflicts is the first step in resolving them. Git will alert you when a conflict occurs. You will see messages like:
CONFLICT (content): Merge conflict in
Automatic merge failed; fix conflicts and then commit the result.
Conflicted files will have markers like:
<<<<<<< HEAD
Your changes
=======
Their changes
>>>>>>> branch-name
Resolving Conflicts
To resolve conflicts, follow these steps:
- Open the conflicted files.
- Look for conflict markers.
- Decide which changes to keep.
- Remove conflict markers after resolving.
- Save the file.
- Commit your changes with:
git add
git commit -m "Resolved conflict in "
Using a merge tool can simplify this process. Popular tools include:
- KDiff3
- Beyond Compare
- WinMerge
Integrate these tools with Git for an easier experience. You can set them up using:
git config --global merge.tool
Best Practices
Forcing a Git pull can be risky if not done correctly. Follow best practices to ensure your codebase remains intact and updated.
Regular Updates
Keep your repository up to date. Regular updates prevent conflicts and make it easier to manage changes.
- Daily Pulls: Pull changes daily to stay current.
- Review Changes: Always review changes before pulling them.
- Automate Updates: Use scripts to automate regular updates.
Backup Strategies
Always have a backup. Backups protect against data loss and make recovery easier.
- Local Backup: Keep a local copy of your work.
- Cloud Backup: Use cloud services for extra security.
- Version Control: Tag stable versions for rollback.
Strategy | Benefit |
---|---|
Local Backup | Quick recovery |
Cloud Backup | Extra security |
Version Control | Easy rollback |
Implementing these best practices will help you manage your Git repository efficiently. Regular updates and backup strategies are essential for maintaining a healthy codebase.
Troubleshooting
Git is a powerful tool for version control. But sometimes, errors happen. It’s common to face issues during a Git pull. Understanding these problems can save time and stress.
Common Errors
During a Git pull, you may encounter various errors. These are common and can be fixed. Here are some frequent issues:
- Merge conflicts: Changes in the local and remote branches differ.
- Authentication errors: Incorrect credentials or expired tokens.
- Detached HEAD: Working in a detached HEAD state.
- Uncommitted changes: Local changes not committed or stashed.
Effective Solutions
Each error has specific solutions. Follow these steps to resolve them.
Merge Conflicts
Merge conflicts occur when changes in local and remote branches differ. To fix:
- Run
git status
to identify conflicting files. - Open conflicting files and edit them.
- After resolving, run
git add
. - Commit the changes with
git commit -m "Resolve conflict"
.
Authentication Errors
Authentication errors often stem from incorrect credentials. To fix:
- Update your credentials or tokens.
- Use
git config --global credential.helper cache
to store credentials.
Detached HEAD
A detached HEAD means working on a specific commit. To fix:
- Run
git checkout master
to return to the main branch. - Use
git branch -d
to delete unnecessary branches.
Uncommitted Changes
Uncommitted changes can block a pull. To fix:
- Commit changes with
git commit -m "Your message"
. - Or, stash changes with
git stash
.
Following these steps ensures a smooth Git pull. Resolve errors quickly and keep coding.
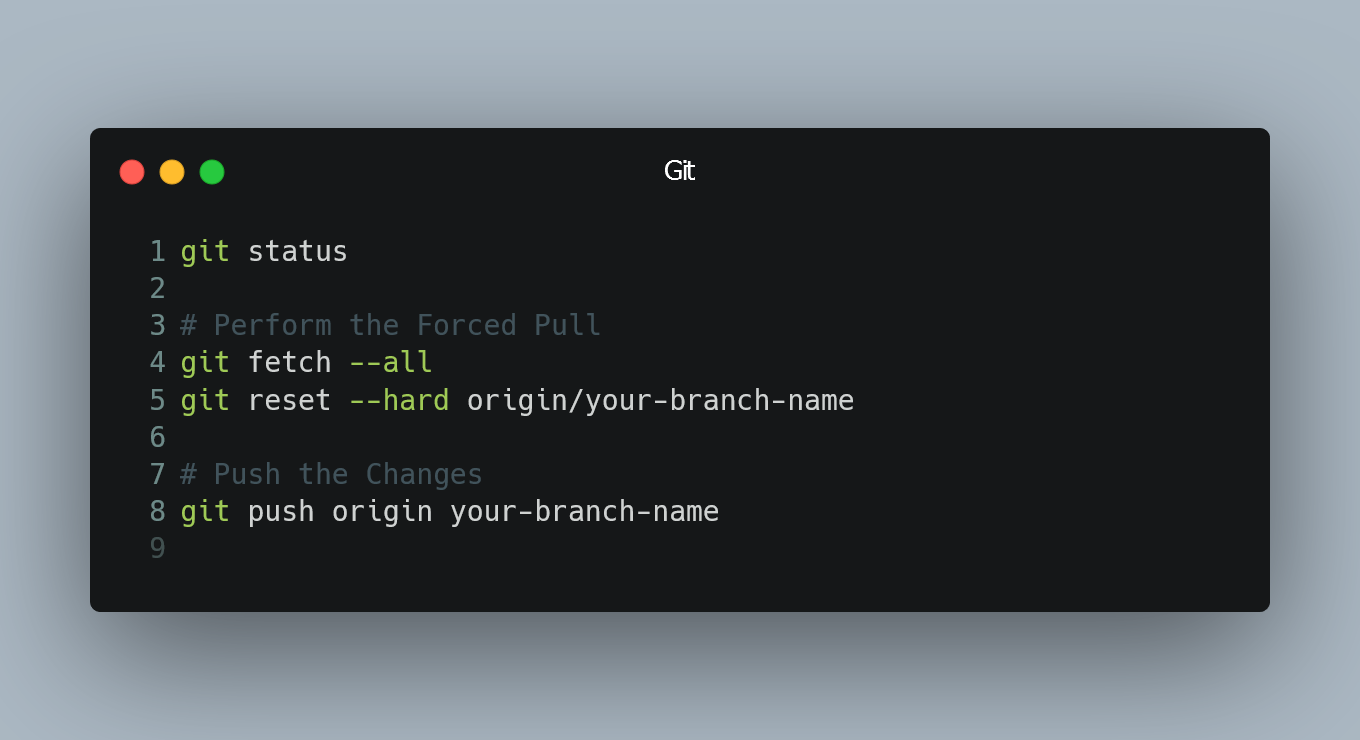
Advanced Tips
In this section, we’ll explore advanced tips for forcing a Git pull. These tips will help streamline your workflow and ensure seamless integration.
Automation Scripts
Automation scripts can simplify your Git pull process. You can use shell scripts or other scripting languages.
Here’s a simple example using a shell script:
#!/bin/bash
git fetch --all
git reset --hard origin/main
git pull origin main
Save this script and run it to automate your Git pull. This ensures your local repo matches the remote.
Benefits of using automation scripts:
- Reduces manual effort
- Ensures consistency
- Minimizes errors
Integrating With Ci/cd
Integrate Git pull commands with CI/CD pipelines to automate deployments. This keeps your codebase up-to-date.
Here’s an example using a YAML file for a CI/CD pipeline:
stages:
- pull
pull:
stage: pull
script:
- git fetch --all
- git reset --hard origin/main
- git pull origin main
This configuration ensures your CI/CD pipeline always has the latest code.
Advantages of CI/CD integration:
- Automates updates
- Ensures code consistency
- Speeds up the deployment process
Frequently Asked Questions
How Do You Force A Git Pull?
To force a git pull, use `git fetch –all` followed by `git reset –hard origin/branch-name`. This will overwrite local changes.
What Happens If You Force A Git Pull?
Forcing a git pull overwrites local changes with the remote repository’s content. Unsaved local changes will be lost.
Can You Force Git Pull Without Losing Changes?
No, forcing a git pull overwrites local changes. Commit or stash changes before forcing a pull to avoid data loss.
How Do I Resolve Conflicts During Git Pull?
To resolve conflicts, use `git status` to identify conflicts. Manually edit conflicting files, then commit the resolved changes.
Conclusion
Mastering the process to force a Git pull is essential for smooth collaboration. It ensures consistent codebases and minimizes conflicts. Practice these steps regularly to enhance your workflow. Remember, a well-synced repository leads to more efficient development. Stay updated with Git techniques for a seamless coding experience.