To force a Git pull, use the command `git fetch –all` followed by `git reset –hard origin/branch-name`. This ensures your local branch matches the remote branch exactly.
Force pulling in Git is a powerful command that helps developers synchronize their local repository with the remote repository. This command is particularly useful when dealing with conflicts or when your local branch has diverged significantly from the remote branch.
By fetching all updates and resetting your local branch, you ensure that your codebase is up-to-date and aligned with the team’s latest changes. Always use this command with caution, as it will overwrite local changes. Understanding how to force pull effectively can streamline your workflow and minimize integration issues.
Introduction To Git Force Pull
Understanding Git is crucial for developers. One important feature is Git Force Pull. It helps keep your codebase synchronized. Let’s explore what it is and why it’s important.
What Is Git Force Pull?
Git Force Pull is a command in Git. It forces a local repository to match the remote repository. Normally, Git merges changes. But with force pull, it overwrites local changes. This ensures you have the latest code.
The command to use is:
git fetch --all
git reset --hard origin/master
This command fetches all updates and then forces the local repository to match the remote repository.
Importance In Development
Keeping codebases synchronized is critical. This avoids conflicts and ensures everyone works on the latest code. Let’s look at why Git Force Pull is important:
- Resolve Conflicts: It helps resolve merge conflicts.
- Consistency: Ensures all team members have the same code.
- Efficiency: Saves time by avoiding manual merges.
In a collaborative environment, these points are essential. They maintain smooth workflows and prevent issues.
Feature | Benefit |
---|---|
Conflict Resolution | Avoids manual conflict resolution. |
Consistency | Ensures uniform code across the team. |
Efficiency | Speeds up the development process. |
Using Git Force Pull wisely can greatly benefit your workflow. It ensures that everyone works with the latest code, reducing errors and improving efficiency.
Understanding Git Basics
Git is a powerful tool for version control. It helps developers manage code. To use Git effectively, you must understand its basics. Let’s explore Git repositories, branches, and merging.
Git Repositories
A Git repository stores your project’s history. It keeps track of every change. You can create a repository using the command:
git init
This command initializes a new repository. You can also clone an existing repository with:
git clone [repository URL]
A cloned repository is a copy of an existing one. You can now work on your project.
Branches And Merging
A branch is a separate line of development. It lets you work on features without affecting the main code. Create a branch with:
git branch [branch-name]
Switch to the new branch using:
git checkout [branch-name]
After making changes, you may want to merge the branch. This integrates your work back into the main branch. Use:
git merge [branch-name]
If there are conflicts, Git will notify you. Resolve them before completing the merge.
When To Use Git Force Pull
Using Git force pull can be a powerful tool. But, it comes with its own risks. This method should be used wisely. Below, we explore the right times to use it.
Conflict Resolution
Conflicts arise when multiple people work on the same file. A Git force pull helps you resolve these conflicts. Here is a step-by-step guide:
- Identify the conflicting files.
- Run
git fetch
to update your repository. - Use
git merge
to see conflicts. - Resolve conflicts manually.
- Run
git add .
to stage resolved files. - Finally, use
git commit
to save changes. - If needed, use
git push --force
to update the remote repository.
Be cautious with the force push command. It overwrites remote changes. Only use it if you are sure.
Updating Local Repositories
Sometimes, your local repository may be out of sync. A force pull can help here. Follow these steps:
- Run
git fetch
to get the latest changes. - Use
git reset --hard origin/main
to update your branch. - Run
git pull
to pull changes from the remote repository. - If needed, use
git pull --force
for a complete update.
This method ensures your local copy matches the remote. It is useful for major updates or resets.
Keep in mind, force pulling will overwrite your local changes. Make sure to backup important work.
Using Git wisely can streamline your development process. Always double-check before using force commands.
Risks And Precautions
Using the Git Force Pull command can sometimes be risky. It can lead to unexpected problems if not used properly. Below are some key risks and precautions you should be aware of.
Data Loss
One major risk is data loss. Force pulling can delete local changes that are not committed.
Consider the following points to avoid data loss:
- Always commit your changes before using force pull.
- Check the status of your working directory.
- Use
git stash
to save changes temporarily.
Overwriting Changes
Another risk is overwriting changes. Force pull can override changes made by your team.
Take these precautions to avoid overwriting changes:
- Communicate with your team before using force pull.
- Check for any recent updates from the remote repository.
- Use
git fetch
andgit merge
commands first.
Risk | Precaution |
---|---|
Data Loss | Commit changes, check status, use git stash |
Overwriting Changes | Communicate, check updates, use git fetch and merge |
Step-by-step Guide
Executing a force pull in Git can be tricky. This guide helps you perform it safely and effectively. Follow these steps to master the Git force pull command.
Command Syntax
To force a pull in Git, you need to use specific commands. The basic syntax is:
git fetch --all
git reset --hard origin/
Here, git fetch --all
updates all branches from the remote. The git reset --hard origin/
command forces your local branch to match the remote branch.
Practical Examples
Let’s look at some practical examples. Assume you are working on the main
branch.
- First, fetch all updates from the remote repository:
git fetch --all
- Next, reset your local branch to match the remote:
git reset --hard origin/main
If you are on a different branch, replace main
with your branch name. For example, for a branch named feature
:
git reset --hard origin/feature
Make sure you have committed or stashed your changes. This operation will overwrite your local changes.
Command | Description |
---|---|
git fetch --all |
Fetches updates from all remote branches. |
git reset --hard origin/ |
Resets local branch to match the remote branch. |
Using these steps, you can perform a Git force pull safely. Always ensure your local changes are backed up before proceeding.
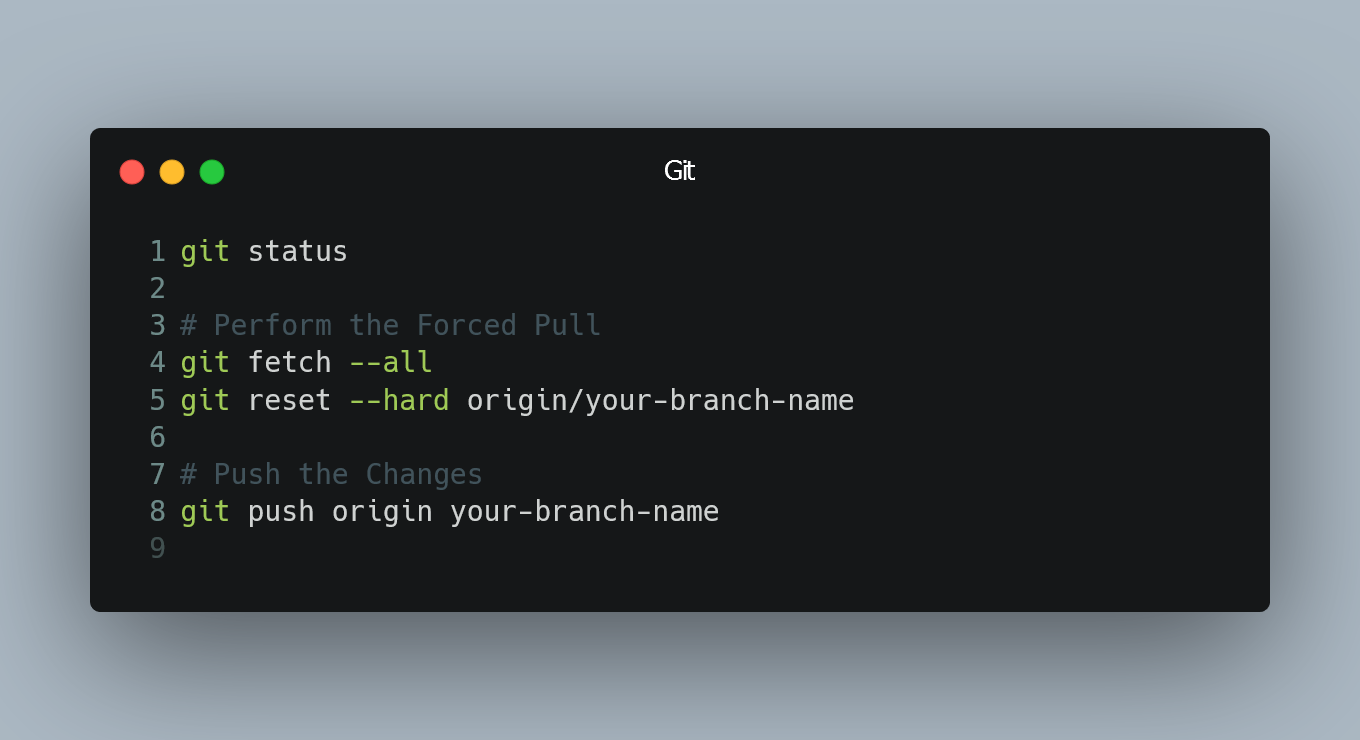
Advanced Techniques
Sometimes, basic Git commands aren’t enough. Advanced techniques offer more control. Let’s explore how to use Git Force Pull for complex tasks.
Rebasing With Force Pull
Rebasing rewrites commit history. It ensures a clean project timeline. Combine it with force pull for powerful results. Here’s how to do it:
- First, fetch the latest changes:
git fetch origin
- Then, rebase your branch:
git rebase origin/main
- If conflicts arise, resolve them manually.
- Finally, force pull the changes:
git pull --force
Use rebasing to keep commits linear. It simplifies the history for all team members.
Cherry-picking Commits
Cherry-picking allows selective commit integration. It is useful for applying specific changes without merging entire branches. Follow these steps:
- Identify the commit hash you need:
git log
- Cherry-pick the commit:
git cherry-pick [commit hash]
- If conflicts occur, resolve them manually.
- Once resolved, use force pull to update:
git pull --force
Cherry-picking is ideal for hotfixes and feature isolation. It gives you precise control over changes.
Common Mistakes
Using Git Force Pull can sometimes lead to mistakes. These mistakes can cause issues in your project. Learn about common errors and how to avoid them.
Ignoring Conflicts
Ignoring conflicts is a major mistake. When you force pull, conflicts may arise. These conflicts need immediate attention.
If ignored, conflicts can break the code. Always check for conflicts and resolve them quickly. Failing to do so can cause project delays.
Misusing Commands
Another common mistake is misusing commands. Using the wrong command can overwrite changes. This can lead to loss of important work.
Here is a table that explains the key differences:
Command | Purpose |
---|---|
git pull |
Fetch and merge changes |
git pull --force |
Forcefully fetch and merge |
Always double-check the command before executing. This simple step can save a lot of trouble.
Remember, using the correct command is crucial. It ensures you don’t lose important data.
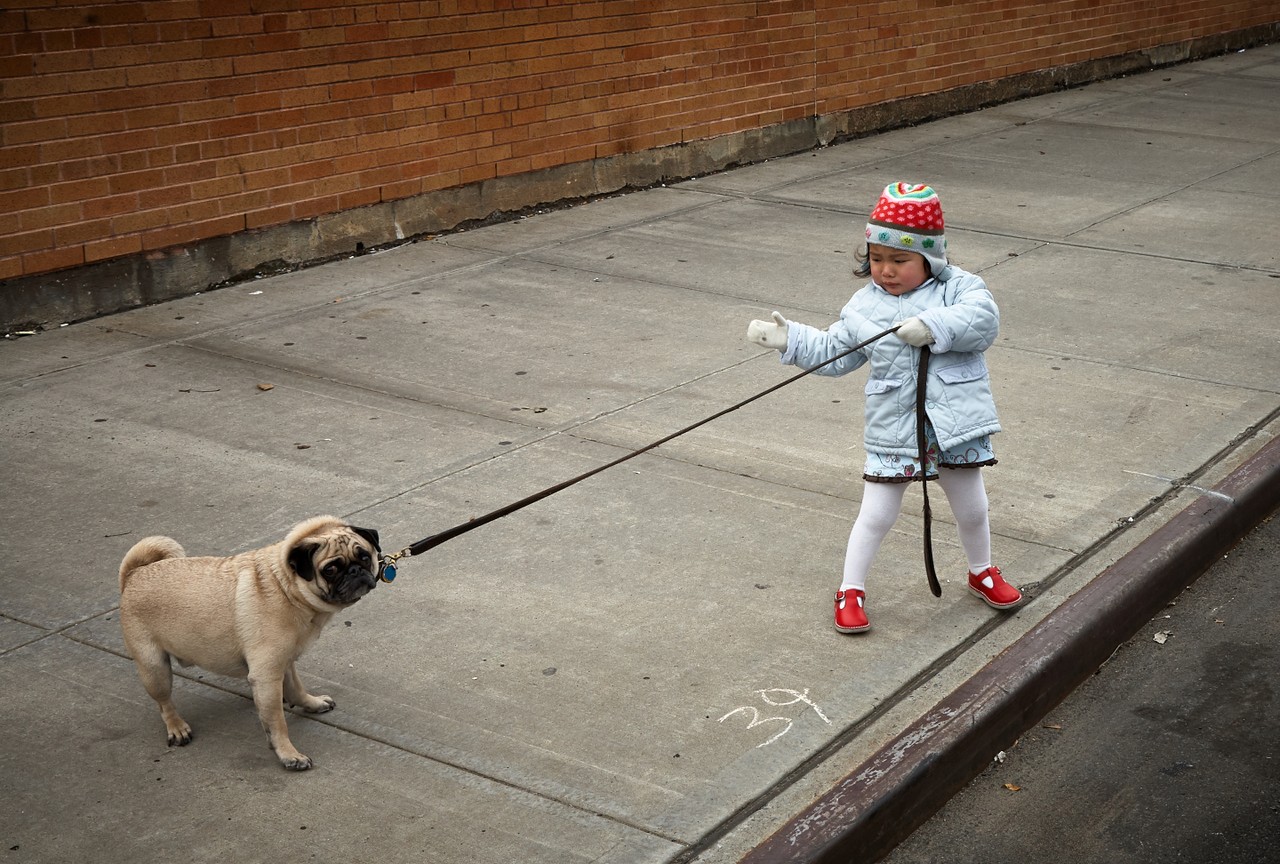
Best Practices
Git force pull is a powerful command. It can overwrite your local changes. So, it’s crucial to follow best practices. This ensures smooth and safe operations. Here, we discuss some of the best practices.
Regular Backups
Before using the git force pull command, make regular backups. This step is essential. It helps you recover lost work.
- Always create a backup branch.
- Use
git branch backup-branch
to create the branch. - Push the branch to remote with
git push origin backup-branch
.
Regular backups prevent data loss. They provide a safety net. This practice is non-negotiable.
Team Communication
Effective communication in your team is vital. Everyone should know about force pulls. This avoids conflicts and confusion.
- Inform your team before force pulling.
- Use team communication tools.
- Document the changes in your project’s README.
Clear communication ensures smooth collaboration. It minimizes risks. Keep your team in the loop always.
Tools And Resources
Git force pull is an essential command for developers. It helps in synchronizing local repositories with remote ones. To make the most of this command, various tools and resources can enhance its functionality.
Useful Plugins
Plugins can significantly boost your Git workflow. Here are some useful plugins:
- GitLens: This plugin provides insights into code changes.
- GitHub Pull Requests: Integrates pull requests directly into your editor.
- Git Graph: Visualizes your repository’s commit history.
- Git Blame: Displays the author of each line of code.
Learning Resources
Understanding Git force pull is crucial. Here are some top learning resources:
Resource | Description |
---|---|
Pro Git Book | An extensive guide to mastering Git. |
GitHub Learning Lab | Interactive tutorials and hands-on projects. |
Codecademy Git Course | Step-by-step lessons on using Git. |
Atlassian Git Tutorials | Comprehensive tutorials from Git basics to advanced topics. |
Utilize these tools and resources to master Git force pull. Enhance your productivity and collaboration.
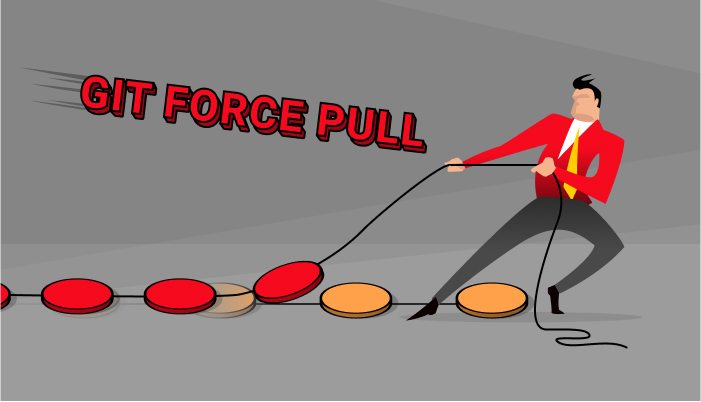
Frequently Asked Questions
What Is A Git Force Pull?
A Git force pull overrides local changes and fetches remote updates. Use it cautiously to avoid losing local work.
How Do You Perform A Git Force Pull?
To perform a Git force pull, use the command `git fetch –all` followed by `git reset –hard origin/branch-name`.
When Should You Use Git Force Pull?
Use Git force pull when your local branch is significantly behind the remote branch and you need a fresh start.
What Are The Risks Of Git Force Pull?
The main risk is losing uncommitted local changes. Always ensure you’ve backed up important work before proceeding.
Conclusion
Mastering the Git force pull command can streamline your workflow. It resolves conflicts and ensures your repository stays updated. Use it wisely to keep your projects in sync. By understanding its power, you can handle complex version control with ease.
Stay productive and maintain clean code with Git force pull.
Leave a Reply
You must be logged in to post a comment.